Getting Started¶
The simplest tasks are pypolycontain
is defining polytopic objects, performing operations, and visualizing them. First, import the package alongside numpy
.
[1]:
import numpy as np
import pypolycontain as pp
Objects¶
H-polytope¶
We define an H-polytope \(\mathbb{P}=\{x \in \mathbb{R}^2 | Hx \le h\}\). We give the following numericals for H and h:
[2]:
H=np.array([[1,1],[-1,1],[0,-1]])
h=np.array([1,1,0])
A=pp.H_polytope(H,h)
This a triangle as it is defined by intersection of 3 half-spaces in \(\mathbb{R}^2\). In order to visualizate the polytope, we call the following function. Note the brackets around visualize
function - it takes in a list of polytopes as its primary argument.
[3]:
pp.visualize([A],title=r'$A$')
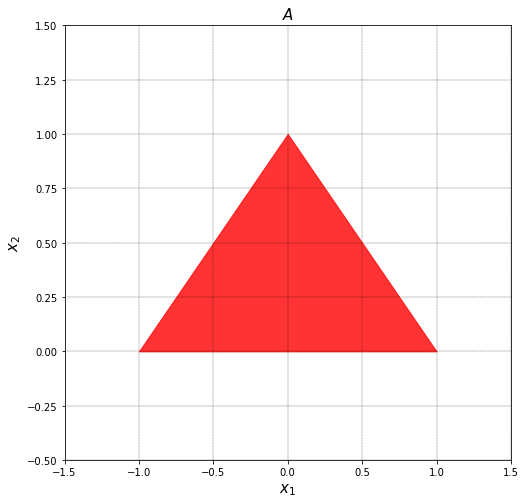
AH-polytope¶
We define an AH-polytope as \(t+T\mathbb{P}\) with the following numbers. The transformation represents a rotation of \(30^\circ\) and translation in \(x\) direction.
[4]:
t=np.array([5,0]).reshape(2,1) # offset
theta=np.pi/6 # 30 degrees
T=np.array([[np.cos(theta),np.sin(theta)],[-np.sin(theta),np.cos(theta)]]) # Linear transformation
B=pp.AH_polytope(t,T,A)
pp.visualize([B],title=r'$B$')
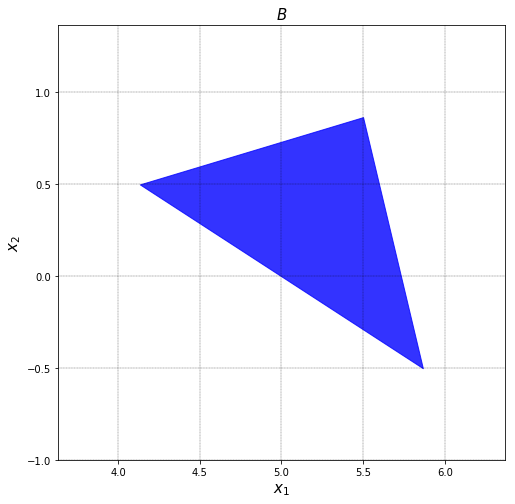
Zonotope¶
We define a zonotope as \(\mathbb{Z}=x+G[-1,1]^{n_p}\), where \(n_p\) is the number of rows in \(p\).
[5]:
x=np.array([4,0]).reshape(2,1) # offset
G=np.array([[1,0,0.5],[0,0.5,-1]]).reshape(2,3)
C=pp.zonotope(x=x,G=G)
pp.visualize([C],title=r'$C$')
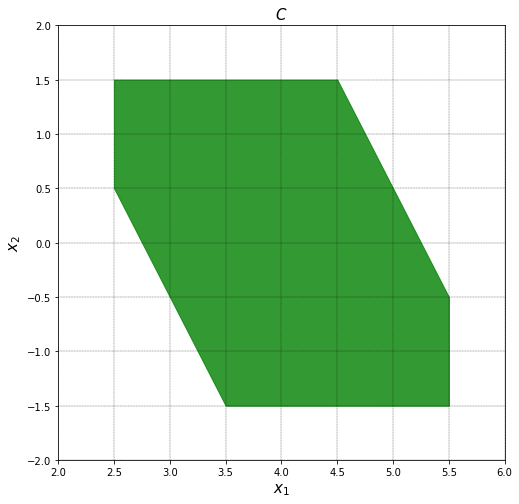
Visualization¶
The visualize
function allows for visualizing multiple polytopic objects.
[6]:
pp.visualize([A,C,B],title=r'$A,B,C$')
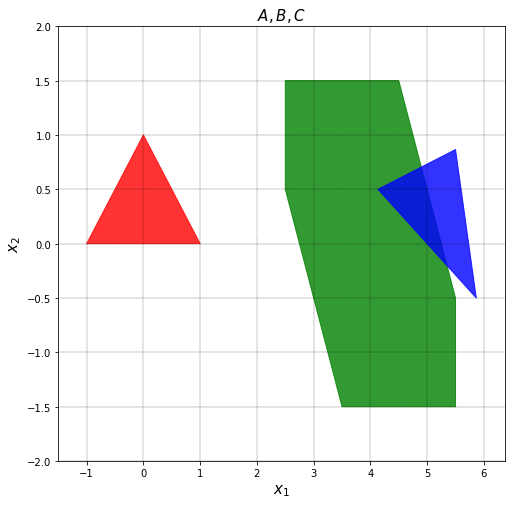
You may have noticed the colors. Here are the default colors for various polytopic objects. Using color argument you can change the color.
Object | Default Color |
---|---|
H-polytope | Red |
Zonotope | Green |
AH-polytope | Blue |
Options¶
visualize
has a set of options. While it only supports 2D plotting, it can take high dimensional polytopes alongside the argument list_of_dimensions
. Its default
is [0,1]
, meaning the projection into the first and second axis is demonstrated.
You can also add a separate subplot
environment to plot the polytopes. Take the following example:
[7]:
import matplotlib.pyplot as plt
fig,ax=plt.subplots()
fig.set_size_inches(6, 3)
pp.visualize([A,B,C],ax=ax,fig=fig)
ax.set_title(r'A triangle (red), rotated by 30 degrees (blue), and a zonotope (green)',FontSize=15)
ax.set_xlabel(r'$x$',FontSize=15)
ax.set_ylabel(r'$y$',FontSize=15)
ax.axis('equal')
[7]:
(-1.343301270189222,
6.209326673973662,
-1.6499999999999997,
1.6499999999999997)
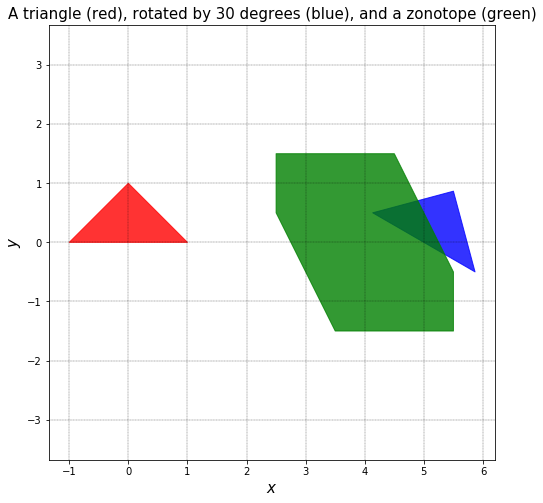
Operations¶
pypolycontain
supports a broad range of polytopic operations. The complete list of operations is here.
Minkowski sum¶
[8]:
D=pp.operations.minkowski_sum(A,B)
D.color=(0.9, 0.9, 0.1)
pp.visualize([D,A,B],title=r'$A$ (red),$B$ (blue), $D=B\oplus C$ (yellow)')
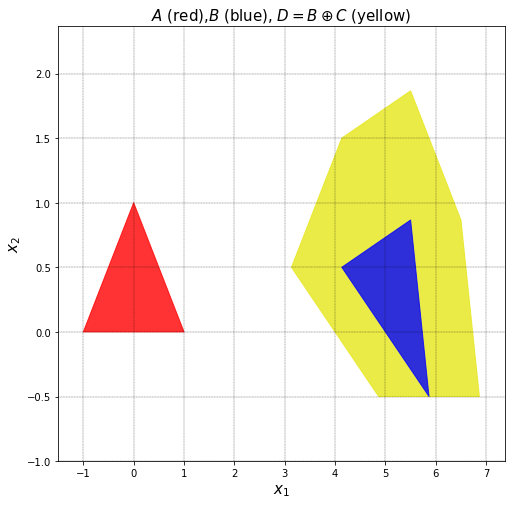
Convex Hull¶
Let’s take the intersection of \(A\) and \(C\).
[9]:
E=pp.convex_hull(A,C)
E.color='purple'
pp.visualize([E,A,C],alpha=0.5,title=r'$A$ (red),$C$ (green), $E={ConvexHull}(A,C)$ (purple)')
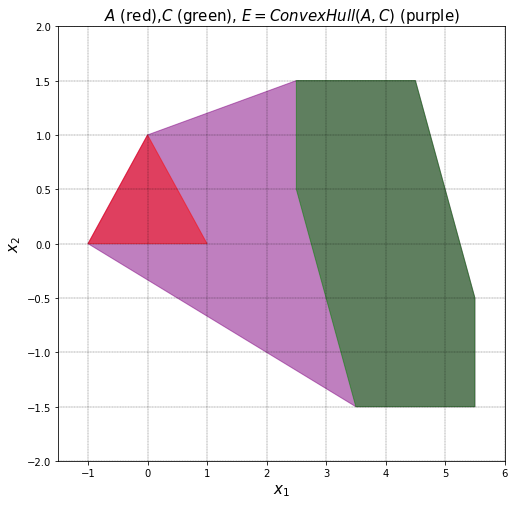
Intersection¶
Let’s take the intersection of \(B\) and \(D\).
[10]:
F=pp.intersection(D,E)
F.color='black'
pp.visualize([D,E,F],alpha=1,title=r'$D$ (yellow), $E$ (purple), $F=D \cap E$ (black)')
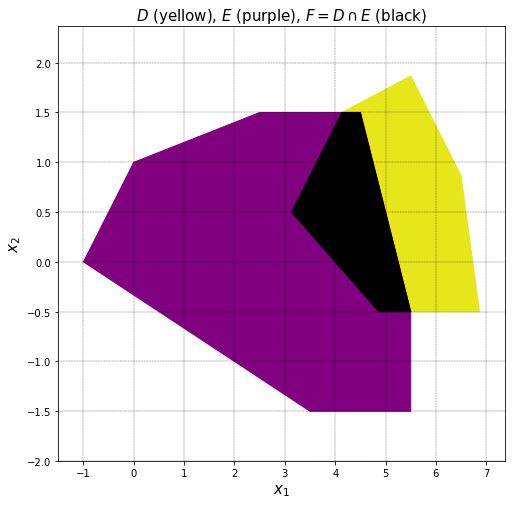
Bounding Box¶
We can compute the bounding box of a polytopic object. For instance, let’s compute the bounding box of \(D\).
[11]:
G=pp.bounding_box(D)
pp.visualize([G,D],title=r'$D$ and its bounding box')
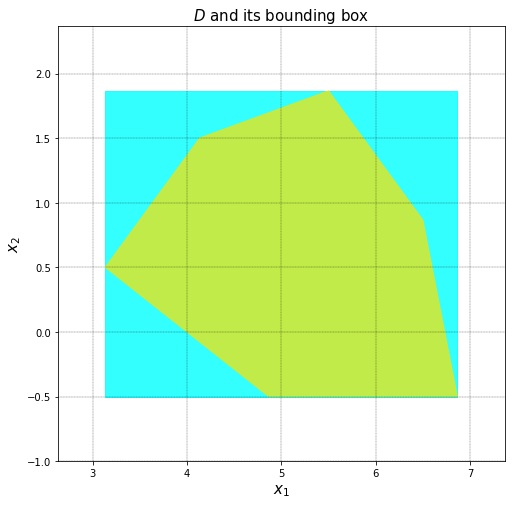
Or the following:
[12]:
mylist=[A,B,C]
pp.visualize([pp.bounding_box(p) for p in mylist]+mylist,title=r'$A,B,C$ and their bounding boxes')
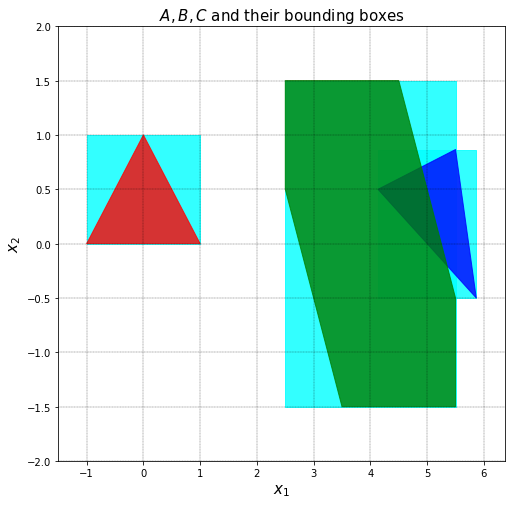